In the previous article, we learnt what the serverless function deployment model was all about. Check out the link below if you missed that article.
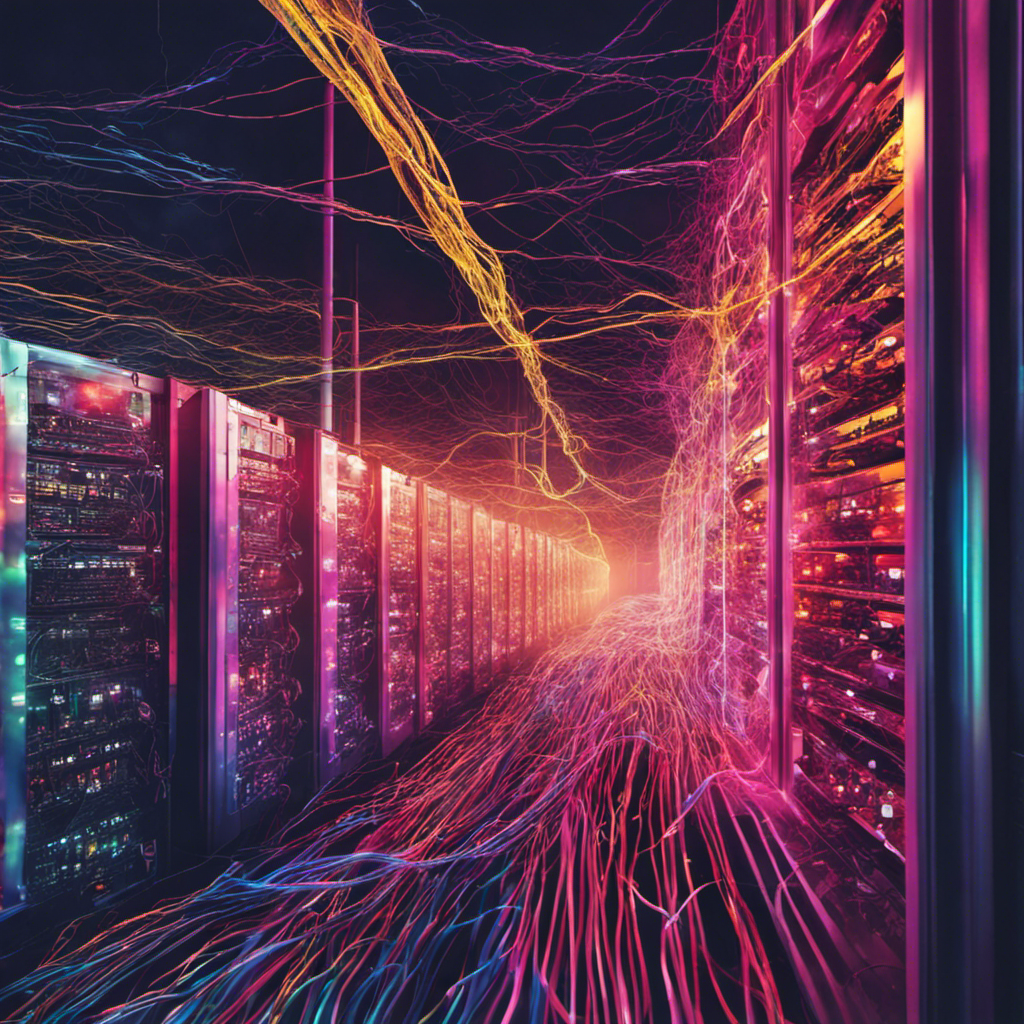
In this article, we will see how to deploy a python app in this model. All the major cloud providers like AWS, Azure and Google Cloud support serverless functions, but it can be quite a challenge to figure out all the steps to get started unless you are an expert in one of those providers. And the pricing can be very obscure.
That's where a whole host of secondary providers have started offering serverless function platforms promising a simple and easy way to deploy on this model.
Today, we are going to look at Vercel's offering. It's a nice one for hobby projects because they have serverless functions available in the free tier. (More on their pricing is available here). I have been using it for about a year for a side project and it's been quite painless, so lets dive right in!
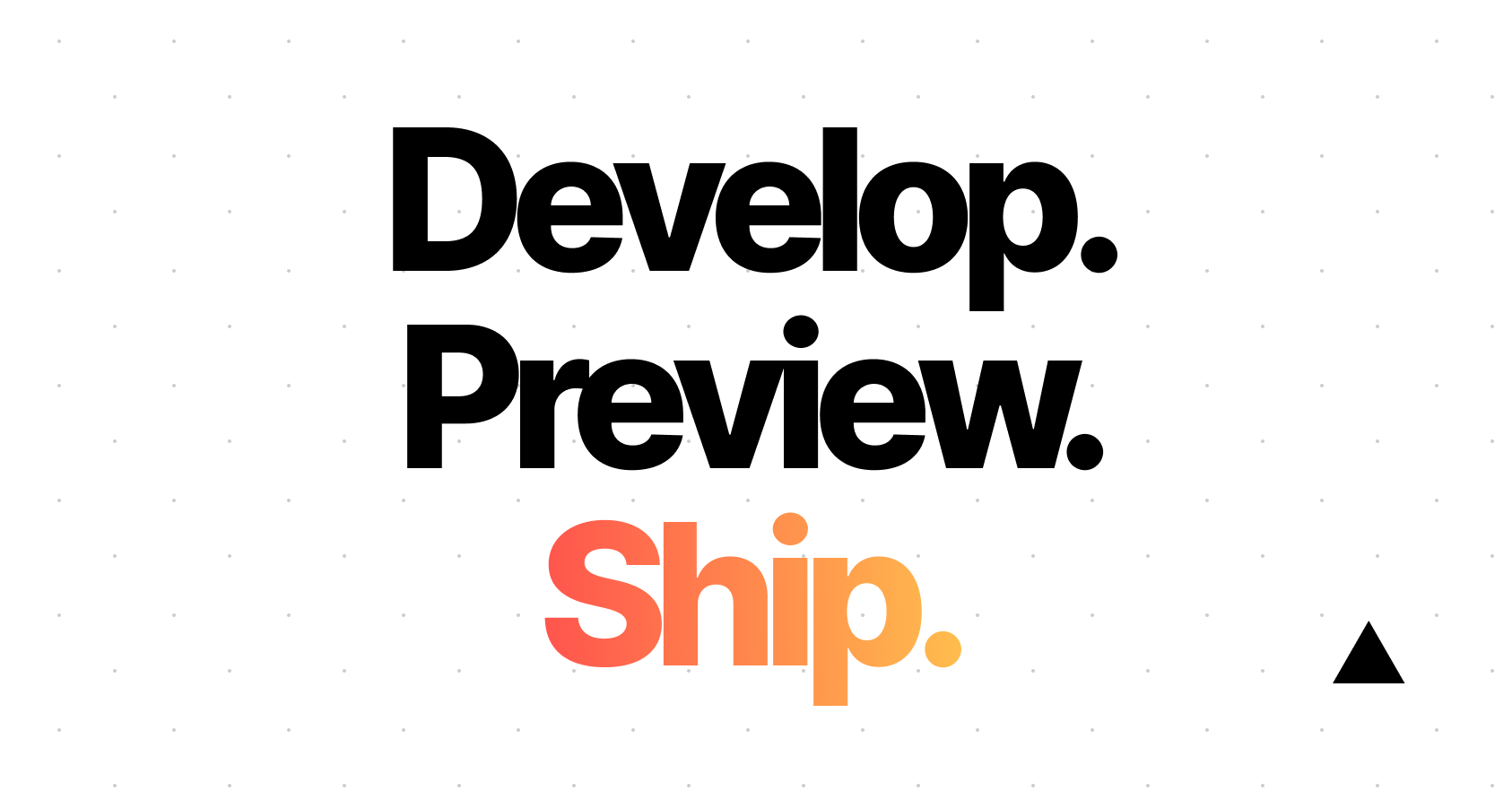
Building the app
Vercel supports both old school Flask and Django apps as well as the newer async frameworks. I decided to go with Starlette for this example. Starlette is one of the older async python web frameworks around and is the base for most of the newer ones like FastAPI. You can learn more about Starlette at the link below
To get started, a folder for the app and initialise a new git repository.
Then create a subfolder called api/
. The api
folder is where we will put all the code for our application.
Now create a file called index.py
in the api
folder and put the following code in there
from starlette.applications import Starlette
from starlette.routing import Route
from starlette.templating import Jinja2Templates
templates = Jinja2Templates(directory='templates')
async def index(request):
return templates.TemplateResponse(
'index.html', {'name': 'World', 'request': request}
)
app = Starlette(routes=[
Route('/', index)
])
This code creates a simple Starlette app. It has one path /
which maps to the index
function. When the path is hit by the client, the function gets executed. All it does is to apply the {'name': 'World'}
data to the index.html
template and return the response.
Next, we need to create the template. If you observe the code above, you can see that we are using the popular Jinja2 library for templating.
Create a folder called templates
in the root of the application. Create a file index.html
there and put the following content for the file
<!DOCTYPE html>
<html>
<head>
<title>My serverless python</title>
</head>
<body>
<h1>Hello {{name}}</h1>
</body>
</html>
This template will take the name
passed into the template context and render it in the heading.
With that, our simple app is now ready for deployment
Preparing for deployment
There are a few steps that we need to take now before we can deploy the application to Vercel. First, we need to create a configuration file.
Create a file called vercel.json
in the root folder of the application, with the following content
{
"routes": [{
"src": "/",
"dest": "/api/index.py"
}]
}
This maps the home page URL to our app
Next, create a requirements.txt
file in the root folder containing the dependencies of the project
starlette==0.27.0
jinja2==3.1.2
Your code structure should have the following layout. Commit and push the code to Github
requirements.json
vercel.json
api/index.py
templates/index.html
Deploying the app
We are now done with all the code. All that is left is to create a project in our Vercel account. Log into Vercel and create a new project by clicking the Add New...
button on the overview page of the Vercel dashboard

The next step is to pull in the code. The simplest way to do that is to add the Vercel app to your github account and give access to the repository you want to deploy. This way Vercel will get notified whenever you push a new change and will automatically redeploy the new version to production.
Once you have selected the repository, you will be asked to configure the project. There are options to customise the settings, but leaving the settings on default should work fine here. It should look like the screen below, apart from the project name being different. The only thing is to ensure that the Framework
field should be set to Other
.
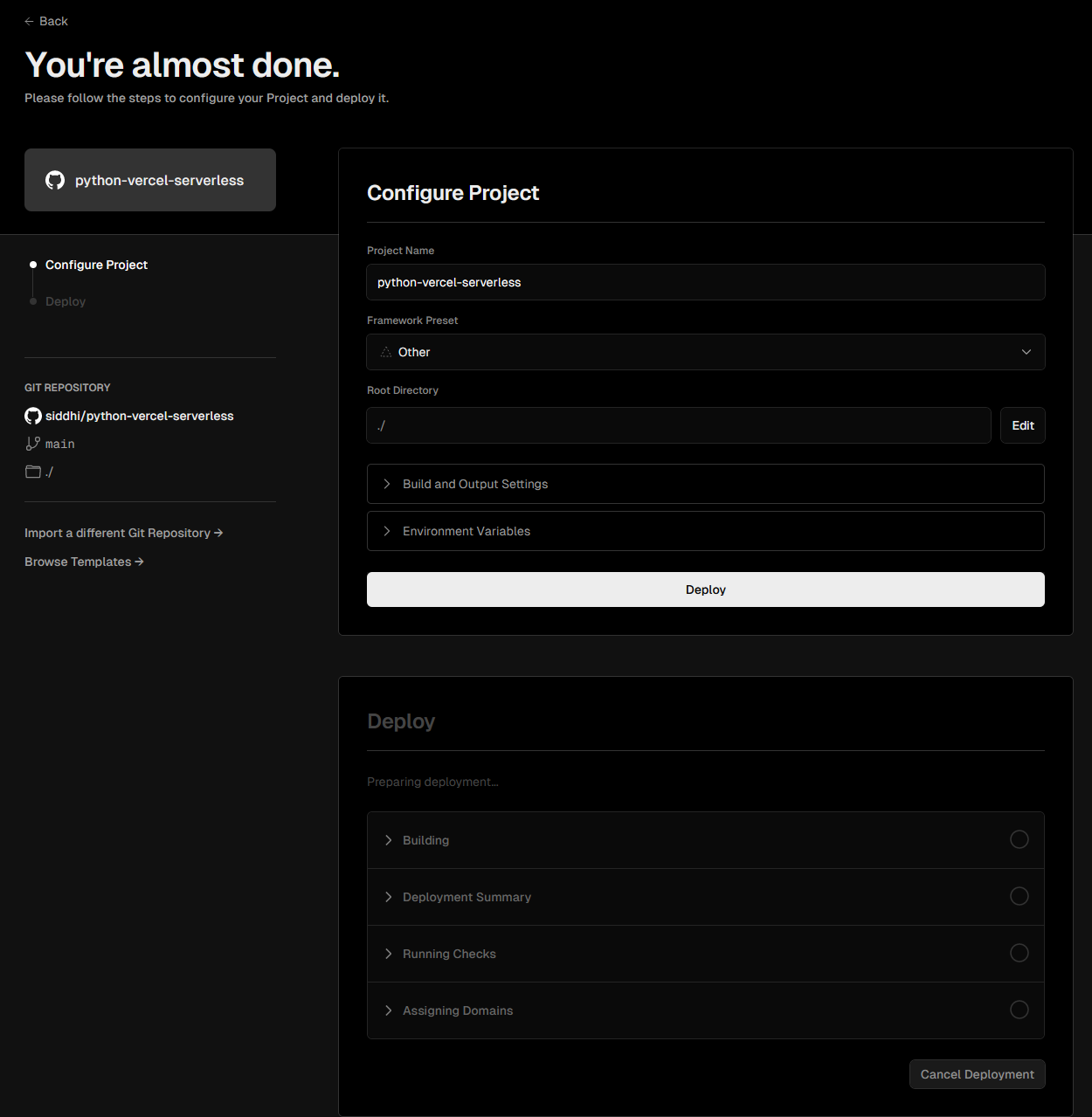
Once you click that deploy button, Vercel will pull in the code from the repository and perform a build and deploy. This should only take a few seconds for our simple app. Vercel will give you some links where you can access the app as well as a screenshot of what the home page looks like.
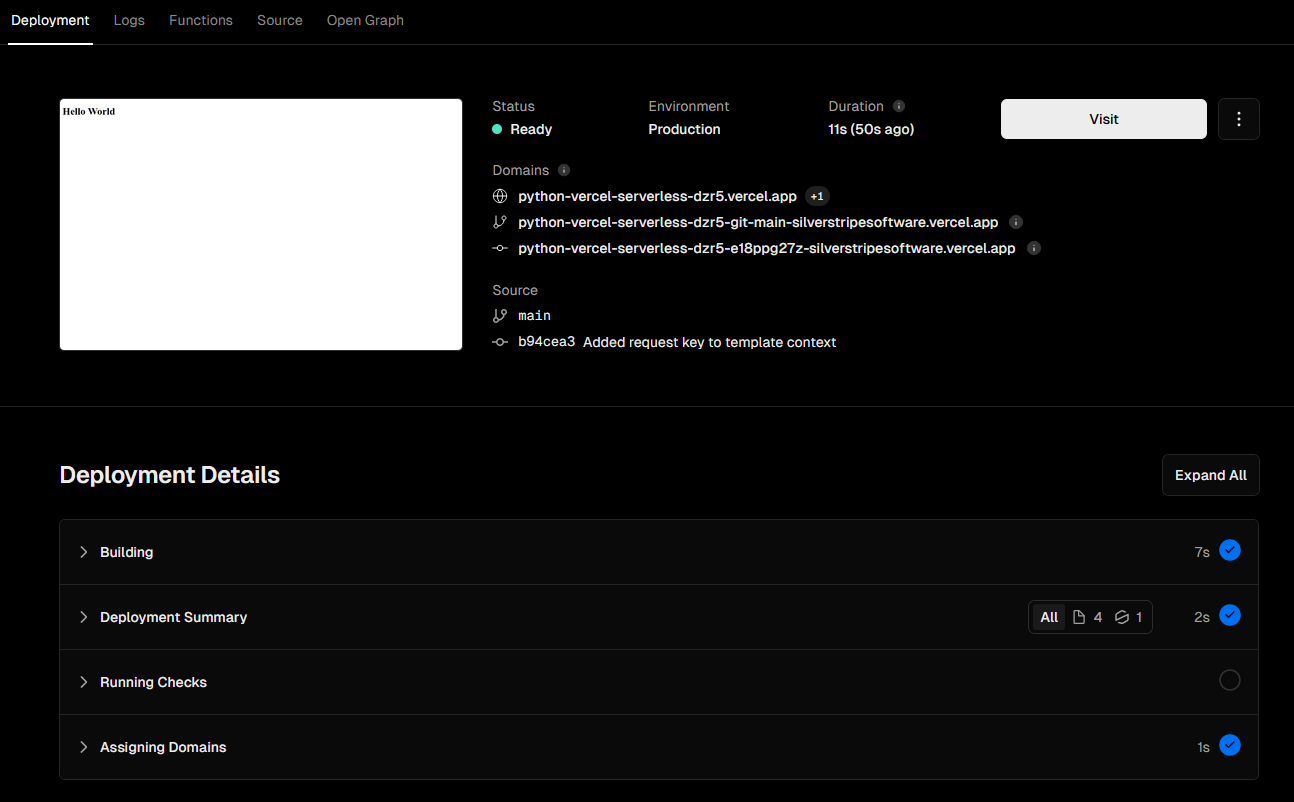
Click one of the links and you should see the app running. Voila!
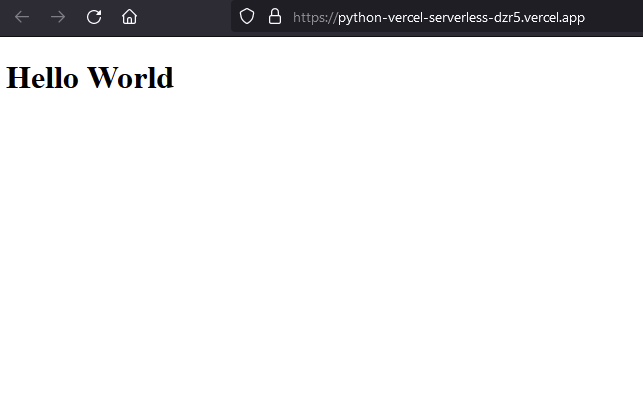
Any time a new commit is pushed, Vercel will automatically pick it up and redeploy the application.
Summary
In this tutorial, we saw how to create a simple 'Hello World' application using the Starlette async web framework and deploy it on Vercel's serverless platform. Now that we have the app deployed on serverless functions, we can use it as a base to build a more complex app.
As explained in the previous article, serverless platform is very useful for projects that have high variability in the traffic load pattern, as well as for hobby projects that do not get a lot of traffic. For applications that receive a steady, predictable stream of traffic, traditional deployment patterns are preferred.
If you would like to get the code for this sample app and fork it for you own use, it is available at the Github like below
Did you like this article?
If you liked this article, consider subscribing to this site. Subscribing is free.
Why subscribe? Here are three reasons:
- You will get every new article as an email in your inbox, so you never miss an article
- You will be able to comment on all the posts, ask questions, etc
- Once in a while, I will be posting conference talk slides, longer form articles (such as this one), and other content as subscriber-only